What Is The Event Handler? How Do I Register/remove The Event Handler For The Node?
Chapter fifteenHandling Events
You accept power over your mind—not outside events. Realize this, and y'all will find force.
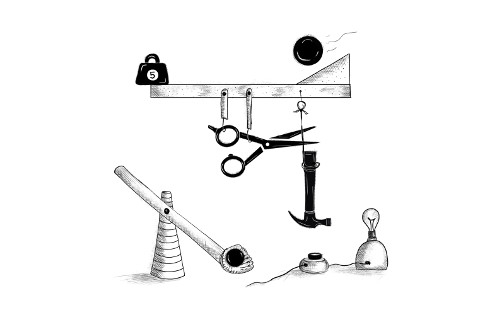
Some programs work with direct user input, such every bit mouse and keyboard actions. That kind of input isn't available as a well-organized information structure—it comes in piece by piece, in real time, and the programme is expected to respond to it as it happens.
Effect handlers
Imagine an interface where the merely fashion to discover out whether a key on the keyboard is existence pressed is to read the current state of that cardinal. To be able to react to keypresses, y'all would have to constantly read the fundamental'south state and then that you'd catch it before it's released again. It would be unsafe to perform other fourth dimension-intensive computations since you lot might miss a keypress.
Some archaic machines exercise handle input like that. A pace up from this would exist for the hardware or operating system to notice the keypress and put it in a queue. A plan can then periodically check the queue for new events and react to what it finds there.
Of form, information technology has to recall to wait at the queue, and to do it frequently, because any time between the key being pressed and the programme noticing the event will cause the software to experience unresponsive. This approach is called polling. Almost programmers prefer to avoid it.
A better mechanism is for the arrangement to actively notify our lawmaking when an event occurs. Browsers practise this past allowing the states to register functions as handlers for specific events.
< p >Click this document to activate the handler.</ p > < script > window.addEventListener("click", () => { console.log("You knocked?"); }); </ script >
The window
binding refers to a built-in object provided past the browser. It represents the browser window that contains the document. Calling its addEventListener
method registers the second argument to be called whenever the event described past its commencement statement occurs.
Events and DOM nodes
Each browser event handler is registered in a context. In the previous example we called addEventListener
on the window
object to register a handler for the whole window. Such a method tin also be institute on DOM elements and some other types of objects. Event listeners are called simply when the event happens in the context of the object they are registered on.
< push button >Click me</ button > < p >No handler here.</ p > < script > permit button = document.querySelector("button"); push.addEventListener("click", () => { panel.log("Button clicked."); }); </ script >
That example attaches a handler to the button node. Clicks on the push button cause that handler to run, but clicks on the residual of the document exercise non.
Giving a node an onclick
attribute has a similar effect. This works for nearly types of events—you can attach a handler through the attribute whose name is the effect name with on
in forepart of information technology.
Merely a node can have but i onclick
attribute, so you tin can annals only one handler per node that fashion. The addEventListener
method allows yous to add any number of handlers so that it is safe to add handlers even if there is already some other handler on the chemical element.
The removeEventListener
method, called with arguments similar to addEventListener
, removes a handler.
< button >Act-once button</ button > < script > let button = certificate.querySelector("button"); role once() { console.log("Done."); push.removeEventListener("click", once); } button.addEventListener("click", once); </ script >
The office given to removeEventListener
has to exist the same part value that was given to addEventListener
. So, to unregister a handler, you'll want to requite the function a name (once
, in the example) to be able to pass the same function value to both methods.
Event objects
Though we have ignored it so far, result handler functions are passed an argument: the effect object. This object holds additional data almost the event. For example, if nosotros want to know which mouse push was pressed, we tin can look at the consequence object's push button
property.
< push button >Click me any fashion you want</ button > < script > let button = document.querySelector("button"); button.addEventListener("mousedown", event => { if (event.button == 0) { console.log("Left push button"); } else if (event.button == 1) { panel.log("Middle button"); } else if (outcome.button == 2) { console.log("Right button"); } }); </ script >
The information stored in an event object differs per type of result. We'll discuss different types later in the chapter. The object'due south type
property always holds a cord identifying the event (such as "click"
or "mousedown"
).
Propagation
For well-nigh effect types, handlers registered on nodes with children volition likewise receive events that happen in the children. If a push inside a paragraph is clicked, event handlers on the paragraph will also run into the click effect.
Merely if both the paragraph and the push have a handler, the more specific handler—the 1 on the push button—gets to go offset. The result is said to propagate outward, from the node where it happened to that node'due south parent node and on to the root of the certificate. Finally, later on all handlers registered on a specific node have had their plow, handlers registered on the whole window get a chance to respond to the event.
At whatever point, an issue handler can phone call the stopPropagation
method on the event object to forestall handlers farther up from receiving the event. This can be useful when, for example, y'all have a button inside another clickable element and you don't desire clicks on the button to activate the outer element's click behavior.
The following example registers "mousedown"
handlers on both a button and the paragraph around it. When clicked with the right mouse button, the handler for the button calls stopPropagation
, which will prevent the handler on the paragraph from running. When the push is clicked with another mouse button, both handlers will run.
< p >A paragraph with a < push button >button</ button >.</ p > < script > permit para = document.querySelector("p"); let button = document.querySelector("button"); para.addEventListener("mousedown", () => { console.log("Handler for paragraph."); }); button.addEventListener("mousedown", event => { console.log("Handler for button."); if (event.button == 2) consequence.stopPropagation(); }); </ script >
Nearly event objects have a target
property that refers to the node where they originated. You can use this holding to ensure that you're not accidentally handling something that propagated up from a node y'all do not want to handle.
It is as well possible to employ the target
holding to bandage a wide net for a specific type of event. For example, if you accept a node containing a long list of buttons, it may exist more user-friendly to register a single click handler on the outer node and take it utilize the target
property to effigy out whether a button was clicked, rather than annals private handlers on all of the buttons.
< button >A</ button > < button >B</ push button > < button >C</ push button > < script > document.torso.addEventListener("click", event => { if (issue.target.nodeName == "Button") { console.log("Clicked", event.target.textContent); } }); </ script >
Default actions
Many events have a default activeness associated with them. If you lot click a link, you will be taken to the link'south target. If you printing the down arrow, the browser volition scroll the page downwards. If you right-click, you lot'll get a context carte du jour. And then on.
For most types of events, the JavaScript event handlers are chosen before the default behavior takes place. If the handler doesn't want this normal behavior to happen, typically because it has already taken care of treatment the event, it can telephone call the preventDefault
method on the event object.
This tin be used to implement your own keyboard shortcuts or context menu. Information technology can also exist used to obnoxiously interfere with the behavior that users expect. For example, hither is a link that cannot be followed:
< a href="https://developer.mozilla.org/" >MDN</ a > < script > let link = document.querySelector("a"); link.addEventListener("click", event => { console.log("Nope."); event.preventDefault(); }); </ script >
Try not to do such things unless y'all have a really good reason to. It'll be unpleasant for people who utilise your folio when expected behavior is broken.
Depending on the browser, some events tin't be intercepted at all. On Chrome, for example, the keyboard shortcut to close the current tab (control-Due west or command-West) cannot be handled by JavaScript.
Fundamental events
When a primal on the keyboard is pressed, your browser fires a "keydown"
event. When information technology is released, you get a "keyup"
consequence.
< p >This folio turns violet when you hold the V key.</ p > < script > window.addEventListener("keydown", upshot => { if (event.key == "v") { document.torso.manner.background = "violet"; } }); window.addEventListener("keyup", consequence => { if (event.fundamental == "v") { document.torso.style.background = ""; } }); </ script >
Despite its name, "keydown"
fires not only when the key is physically pushed down. When a key is pressed and held, the event fires over again every time the cardinal repeats. Sometimes you have to be conscientious well-nigh this. For example, if you add together a button to the DOM when a key is pressed and remove information technology again when the key is released, yous might accidentally add hundreds of buttons when the key is held downward longer.
The example looked at the central
holding of the outcome object to encounter which key the event is almost. This property holds a string that, for most keys, corresponds to the thing that pressing that fundamental would type. For special keys such as enter, it holds a cord that names the key ("Enter"
, in this instance). If you agree shift while pressing a cardinal, that might also influence the proper noun of the key—"v"
becomes "V"
, and "1"
may become "!"
, if that is what pressing shift-ane produces on your keyboard.
Modifier keys such as shift, control, alt, and meta (command on Mac) generate central events but similar normal keys. Only when looking for key combinations, you can also detect out whether these keys are held down by looking at the shiftKey
, ctrlKey
, altKey
, and metaKey
properties of keyboard and mouse events.
< p >Printing Command-Space to continue.</ p > < script > window.addEventListener("keydown", event => { if (event.key == " " & & event.ctrlKey) { console.log("Continuing!"); } }); </ script >
The DOM node where a key event originates depends on the element that has focus when the key is pressed. Most nodes cannot have focus unless you give them a tabindex
aspect, just things similar links, buttons, and form fields can. We'll come back to form fields in Affiliate 18. When nothing in particular has focus, certificate.body
acts as the target node of key events.
When the user is typing text, using fundamental events to figure out what is being typed is problematic. Some platforms, almost notably the virtual keyboard on Android phones, don't fire key events. But fifty-fifty when yous take an old-fashioned keyboard, some types of text input don't match key presses in a straightforward way, such as input method editor (IME) software used by people whose scripts don't fit on a keyboard, where multiple central strokes are combined to create characters.
To discover when something was typed, elements that you tin blazon into, such as the <input>
and <textarea>
tags, burn "input"
events whenever the user changes their content. To get the actual content that was typed, it is all-time to direct read it from the focused field. Chapter 18 will testify how.
Arrow events
At that place are currently 2 widely used ways to bespeak at things on a screen: mice (including devices that act like mice, such every bit touchpads and trackballs) and touchscreens. These produce different kinds of events.
Mouse clicks
Pressing a mouse button causes a number of events to fire. The "mousedown"
and "mouseup"
events are like to "keydown"
and "keyup"
and fire when the button is pressed and released. These happen on the DOM nodes that are immediately below the mouse pointer when the event occurs.
Subsequently the "mouseup"
consequence, a "click"
event fires on the almost specific node that contained both the printing and the release of the push. For case, if I printing down the mouse button on ane paragraph and then move the arrow to another paragraph and release the push, the "click"
issue will happen on the element that contains both those paragraphs.
If ii clicks happen close together, a "dblclick"
(double-click) event also fires, subsequently the second click event.
To get precise information most the place where a mouse event happened, you can wait at its clientX
and clientY
properties, which incorporate the event'due south coordinates (in pixels) relative to the top-left corner of the window, or pageX
and pageY
, which are relative to the pinnacle-left corner of the whole document (which may be different when the window has been scrolled).
The following implements a primitive drawing programme. Every time yous click the certificate, it adds a dot under your mouse pointer. See Affiliate 19 for a less primitive drawing program.
< way > trunk { height: 200px; groundwork: beige; } .dot { height: 8px; width: 8px; edge-radius: 4px; background: blue; position: absolute; } </ manner > < script > window.addEventListener("click", outcome => { let dot = document.createElement("div"); dot.className = "dot"; dot.mode.left = (event.pageX - 4) + "px"; dot.way.top = (consequence.pageY - 4) + "px"; document.body.appendChild(dot); }); </ script >
Mouse motion
Every time the mouse pointer moves, a "mousemove"
event is fired. This effect tin can exist used to track the position of the mouse. A common state of affairs in which this is useful is when implementing some form of mouse-dragging functionality.
Equally an example, the following plan displays a bar and sets up event handlers so that dragging to the left or right on this bar makes it narrower or wider:
< p >Drag the bar to change its width:</ p > < div way="background: orange; width: 60px; peak: 20px" > </ div > < script > let lastX; let bar = certificate.querySelector("div"); bar.addEventListener("mousedown", event => { if (outcome.push == 0) { lastX = upshot.clientX; window.addEventListener("mousemove", moved); event.preventDefault(); } }); role moved(result) { if (effect.buttons == 0) { window.removeEventListener("mousemove", moved); } else { let dist = event.clientX - lastX; allow newWidth = Math.max(10, bar.offsetWidth + dist); bar.style.width = newWidth + "px"; lastX = event.clientX; } } </ script >
Note that the "mousemove"
handler is registered on the whole window. Even if the mouse goes outside of the bar during resizing, every bit long every bit the button is held nosotros even so want to update its size.
We must finish resizing the bar when the mouse push is released. For that, we tin can use the buttons
belongings (notation the plural), which tells us nigh the buttons that are currently held down. When this is zero, no buttons are downward. When buttons are held, its value is the sum of the codes for those buttons—the left button has code one, the right button 2, and the middle one iv. With the left and correct buttons held, for example, the value of buttons
will be iii.
Annotation that the order of these codes is different from the one used past button
, where the centre button came before the correct one. As mentioned, consistency isn't really a stiff point of the browser's programming interface.
Touch on events
The style of graphical browser that nosotros apply was designed with mouse interfaces in heed, at a time where touchscreens were rare. To make the Web "piece of work" on early touchscreen phones, browsers for those devices pretended, to a certain extent, that touch events were mouse events. If you lot tap your screen, yous'll go "mousedown"
, "mouseup"
, and "click"
events.
But this illusion isn't very robust. A touchscreen works differently from a mouse: it doesn't have multiple buttons, you can't track the finger when information technology isn't on the screen (to simulate "mousemove"
), and information technology allows multiple fingers to exist on the screen at the same time.
Mouse events cover touch interaction only in straightforward cases—if y'all add together a "click"
handler to a button, touch users will yet exist able to use it. But something like the resizeable bar in the previous instance does not piece of work on a touchscreen.
There are specific consequence types fired by touch on interaction. When a finger starts touching the screen, you get a "touchstart"
consequence. When information technology is moved while touching, "touchmove"
events fire. Finally, when it stops touching the screen, you lot'll see a "touchend"
event.
Because many touchscreens can detect multiple fingers at the same time, these events don't have a single set of coordinates associated with them. Rather, their consequence objects have a touches
property, which holds an array-like object of points, each of which has its own clientX
, clientY
, pageX
, and pageY
properties.
You could practice something like this to bear witness carmine circles effectually every touching finger:
< style > dot { position: accented; brandish: block; edge: 2px solid red; border-radius: 50px; pinnacle: 100px; width: 100px; } </ style > < p >Touch this page</ p > < script > function update(outcome) { for (let dot; dot = document.querySelector("dot");) { dot.remove(); } for (let i = 0; i < event.touches.length; i ++) { let {pageX, pageY} = consequence.touches[i]; allow dot = document.createElement("dot"); dot.style.left = (pageX - fifty) + "px"; dot.style.top = (pageY - 50) + "px"; certificate.torso.appendChild(dot); } } window.addEventListener("touchstart", update); window.addEventListener("touchmove", update); window.addEventListener("touchend", update); </ script >
You'll often want to call preventDefault
in touch consequence handlers to override the browser's default behavior (which may include scrolling the folio on swiping) and to prevent the mouse events from being fired, for which you may besides take a handler.
Scroll events
Whenever an chemical element is scrolled, a "ringlet"
result is fired on it. This has various uses, such as knowing what the user is currently looking at (for disabling off-screen animations or sending spy reports to your evil headquarters) or showing some indication of progress (by highlighting part of a tabular array of contents or showing a page number).
The following instance draws a progress bar above the document and updates information technology to fill up as you curlicue downwards:
< style > #progress { border-bottom: 2px solid bluish; width: 0; position: fixed; top: 0; left: 0; } </ way > < div id="progress" > </ div > < script > certificate.trunk.appendChild(document.createTextNode( "supercalifragilisticexpialidocious ".echo(1000))); let bar = certificate.querySelector("#progress"); window.addEventListener("coil", () => { let max = document.trunk.scrollHeight - innerHeight; bar.mode.width = `${(pageYOffset / max) * 100 } %`; }); </ script >
Giving an element a position
of fixed
acts much like an absolute
position but also prevents it from scrolling along with the residue of the certificate. The outcome is to make our progress bar stay at the acme. Its width is changed to indicate the electric current progress. Nosotros use %
, rather than px
, as a unit of measurement when setting the width so that the element is sized relative to the page width.
The global innerHeight
binding gives us the height of the window, which we have to decrease from the total scrollable height—yous can't keep scrolling when you hit the bottom of the document. There'due south also an innerWidth
for the window width. By dividing pageYOffset
, the current scroll position, by the maximum whorl position and multiplying by 100, we get the percentage for the progress bar.
Calling preventDefault
on a roll effect does non preclude the scrolling from happening. In fact, the event handler is chosen only after the scrolling takes place.
Focus events
When an element gains focus, the browser fires a "focus"
event on it. When it loses focus, the element gets a "blur"
result.
Unlike the events discussed earlier, these two events exercise not propagate. A handler on a parent element is not notified when a child element gains or loses focus.
The following instance displays assistance text for the text field that currently has focus:
< p >Proper name: < input type="text" data-help="Your full name" > </ p > < p >Historic period: < input type="text" data-help="Your age in years" > </ p > < p id="help" > </ p > < script > let help = certificate.querySelector("#assist"); let fields = document.querySelectorAll("input"); for (let field of Array.from(fields)) { field.addEventListener("focus", event => { allow text = issue.target.getAttribute("data-help"); help.textContent = text; }); field.addEventListener("blur", consequence => { help.textContent = ""; }); } </ script >
The window object will receive "focus"
and "blur"
events when the user moves from or to the browser tab or window in which the certificate is shown.
Load consequence
When a page finishes loading, the "load"
effect fires on the window and the document torso objects. This is often used to schedule initialization deportment that require the whole document to have been congenital. Call up that the content of <script>
tags is run immediately when the tag is encountered. This may exist as well shortly, for example when the script needs to practice something with parts of the certificate that appear later the <script>
tag.
Elements such as images and script tags that load an external file also have a "load"
outcome that indicates the files they reference were loaded. Similar the focus-related events, loading events exercise not propagate.
When a folio is closed or navigated abroad from (for example, by post-obit a link), a "beforeunload"
event fires. The master use of this event is to prevent the user from accidentally losing work past closing a document. If you foreclose the default behavior on this event and set up the returnValue
holding on the event object to a string, the browser will testify the user a dialog request if they actually desire to go out the page. That dialog might include your string, but because some malicious sites try to use these dialogs to misfile people into staying on their page to look at dodgy weight loss ads, near browsers no longer brandish them.
Events and the issue loop
In the context of the upshot loop, every bit discussed in Chapter 11, browser event handlers comport like other asynchronous notifications. They are scheduled when the event occurs simply must expect for other scripts that are running to end earlier they get a take chances to run.
The fact that events can be processed just when nothing else is running means that, if the event loop is tied up with other piece of work, any interaction with the folio (which happens through events) will be delayed until there's fourth dimension to procedure it. So if yous schedule likewise much work, either with long-running event handlers or with lots of short-running ones, the folio will become deadening and cumbersome to use.
For cases where you lot actually do want to do some fourth dimension-consuming thing in the background without freezing the folio, browsers provide something called web workers. A worker is a JavaScript process that runs alongside the master script, on its own timeline.
Imagine that squaring a number is a heavy, long-running ciphering that we want to perform in a separate thread. We could write a file called code/
that responds to messages by calculating a foursquare and sending a message back.
addEventListener("message", upshot => { postMessage(event.data * event.data); });
To avoid the issues of having multiple threads touching the aforementioned data, workers do not share their global scope or any other data with the master script's environment. Instead, you have to communicate with them by sending letters back and forth.
This lawmaking spawns a worker running that script, sends information technology a few letters, and outputs the responses.
let squareWorker = new Worker("code/squareworker.js"); squareWorker.addEventListener("message", event => { console.log("The worker responded:", result.data); }); squareWorker.postMessage(ten); squareWorker.postMessage(24);
The postMessage
function sends a message, which volition cause a "bulletin"
issue to burn down in the receiver. The script that created the worker sends and receives messages through the Worker
object, whereas the worker talks to the script that created information technology by sending and listening straight on its global scope. Simply values that can be represented as JSON tin can be sent equally messages—the other side will receive a copy of them, rather than the value itself.
Timers
Nosotros saw the setTimeout
role in Chapter 11. Information technology schedules another function to be called later, after a given number of milliseconds.
Sometimes you demand to cancel a part y'all take scheduled. This is done by storing the value returned by setTimeout
and calling clearTimeout
on it.
let bombTimer = setTimeout(() => { console.log("Smash!"); }, 500); if (Math.random() < 0.5) { console.log("Defused."); clearTimeout(bombTimer); }
The cancelAnimationFrame
part works in the aforementioned way every bit clearTimeout
—calling it on a value returned past requestAnimationFrame
volition cancel that frame (assuming information technology hasn't already been called).
A like prepare of functions, setInterval
and clearInterval
, are used to set timers that should repeat every 10 milliseconds.
let ticks = 0; let clock = setInterval(() => { console.log("tick", ticks ++); if (ticks == 10) { clearInterval(clock); console.log("stop."); } }, 200);
Debouncing
Some types of events take the potential to burn down speedily, many times in a row (the "mousemove"
and "gyre"
events, for example). When handling such events, you must exist careful not to do annihilation too time-consuming or your handler will take upward so much time that interaction with the certificate starts to experience slow.
If you exercise need to do something nontrivial in such a handler, you can use setTimeout
to make sure you are non doing it too often. This is usually called debouncing the consequence. There are several slightly unlike approaches to this.
In the commencement example, we want to react when the user has typed something, but we don't want to do it immediately for every input result. When they are typing chop-chop, we just want to wait until a pause occurs. Instead of immediately performing an action in the event handler, we set a timeout. We also clear the previous timeout (if whatever) so that when events occur close together (closer than our timeout delay), the timeout from the previous effect will exist canceled.
< textarea >Type something hither...</ textarea > < script > let textarea = document.querySelector("textarea"); let timeout; textarea.addEventListener("input", () => { clearTimeout(timeout); timeout = setTimeout(() => console.log("Typed!"), 500); }); </ script >
Giving an undefined value to clearTimeout
or calling it on a timeout that has already fired has no consequence. Thus, we don't accept to exist careful about when to call it, and we simply do so for every issue.
We can use a slightly different pattern if we want to space responses so that they're separated by at least a certain length of time just want to burn them during a series of events, not but afterward. For example, we might want to respond to "mousemove"
events by showing the current coordinates of the mouse but but every 250 milliseconds.
< script > let scheduled = null; window.addEventListener("mousemove", event => { if (! scheduled) { setTimeout(() => { document.trunk.textContent = `Mouse at ${ scheduled.pageX } , ${ scheduled.pageY } `; scheduled = null; }, 250); } scheduled = event; }); </ script >
Summary
Event handlers make information technology possible to observe and react to events happening in our web page. The addEventListener
method is used to register such a handler.
Each event has a type ("keydown"
, "focus"
, and so on) that identifies it. Most events are called on a specific DOM chemical element and then propagate to that element's ancestors, allowing handlers associated with those elements to handle them.
When an effect handler is called, it is passed an event object with boosted information well-nigh the outcome. This object likewise has methods that allow united states of america to stop farther propagation (stopPropagation
) and prevent the browser's default handling of the event (preventDefault
).
Pressing a key fires "keydown"
and "keyup"
events. Pressing a mouse button fires "mousedown"
, "mouseup"
, and "click"
events. Moving the mouse fires "mousemove"
events. Touchscreen interaction will result in "touchstart"
, "touchmove"
, and "touchend"
events.
Scrolling tin exist detected with the "roll"
issue, and focus changes can exist detected with the "focus"
and "mistiness"
events. When the certificate finishes loading, a "load"
event fires on the window.
Exercises
Balloon
Write a page that displays a balloon (using the balloon emoji, 🎈). When you press the upwardly pointer, information technology should inflate (grow) 10 percent, and when you lot press the down arrow, it should deflate (compress) 10 percent.
You can control the size of text (emoji are text) by setting the font-size
CSS property (style.fontSize
) on its parent element. Think to include a unit in the value—for instance, pixels (10px
).
The primal names of the arrow keys are "ArrowUp"
and "ArrowDown"
. Make sure the keys change simply the balloon, without scrolling the folio.
When that works, add a feature where, if you accident up the airship past a certain size, it explodes. In this case, exploding means that it is replaced with an 💥 emoji, and the event handler is removed (so that you tin can't inflate or debunk the explosion).
< p >🎈</ p > < script > </ script >
You'll desire to annals a handler for the "keydown"
event and wait at event.cardinal
to figure out whether the upwardly or downward arrow key was pressed.
The current size tin be kept in a bounden then that yous can base the new size on it. It'll be helpful to define a office that updates the size—both the binding and the way of the balloon in the DOM—so that you can call it from your event handler, and possibly as well once when starting, to set the initial size.
You can modify the airship to an explosion by replacing the text node with another one (using replaceChild
) or by setting the textContent
belongings of its parent node to a new string.
Mouse trail
In JavaScript's early days, which was the high fourth dimension of gaudy home pages with lots of animated images, people came up with some truly inspiring ways to employ the language.
One of these was the mouse trail—a series of elements that would follow the mouse pointer as you moved it across the page.
In this exercise, I want you to implement a mouse trail. Use admittedly positioned <div>
elements with a fixed size and background color (refer to the code in the "Mouse Clicks" department for an example). Create a bunch of such elements and, when the mouse moves, brandish them in the wake of the mouse pointer.
There are diverse possible approaches hither. You can make your solution as simple or equally complex every bit you desire. A elementary solution to offset with is to keep a fixed number of trail elements and cycle through them, moving the next one to the mouse's current position every time a "mousemove"
outcome occurs.
< style > .trail { position: absolute; superlative: 6px; width: 6px; edge-radius: 3px; background: teal; } body { acme: 300px; } </ fashion > < script > </ script >
Creating the elements is best washed with a loop. Append them to the document to brand them show up. To be able to access them later to alter their position, you lot'll want to shop the elements in an array.
Cycling through them can be done by keeping a counter variable and adding i to information technology every fourth dimension the "mousemove"
outcome fires. The remainder operator (% elements.
) can so exist used to get a valid array alphabetize to pick the element y'all desire to position during a given event.
Some other interesting result can exist achieved past modeling a simple physics system. Employ the "mousemove"
event only to update a pair of bindings that rail the mouse position. So use requestAnimationFrame
to simulate the trailing elements being attracted to the position of the mouse arrow. At every animation step, update their position based on their position relative to the pointer (and, optionally, a speed that is stored for each element). Figuring out a skillful style to exercise this is upwardly to you lot.
Tabs
Tabbed panels are widely used in user interfaces. They allow you to select an interface panel past choosing from a number of tabs "sticking out" above an element.
In this exercise you lot must implement a simple tabbed interface. Write a function, asTabs
, that takes a DOM node and creates a tabbed interface showing the child elements of that node. It should insert a listing of <button>
elements at the top of the node, one for each child element, containing text retrieved from the data-tabname
attribute of the kid. All but ane of the original children should be subconscious (given a brandish
style of none
). The currently visible node tin can be selected by clicking the buttons.
When that works, extend it to style the push button for the currently selected tab differently so that it is obvious which tab is selected.
< tab-console > < div information-tabname="one" >Tab one</ div > < div data-tabname="two" >Tab two</ div > < div data-tabname="3" >Tab iii</ div > </ tab-panel > < script > function asTabs(node) { } asTabs(document.querySelector("tab-console")); </ script >
One pitfall you lot might run into is that you tin't directly utilise the node's childNodes
property equally a collection of tab nodes. For i thing, when yous add together the buttons, they will also become child nodes and end up in this object because it is a live data structure. For another, the text nodes created for the whitespace between the nodes are too in childNodes
just should not become their own tabs. You can use children
instead of childNodes
to ignore text nodes.
You could start by building up an array of tabs and then that you have like shooting fish in a barrel admission to them. To implement the styling of the buttons, yous could store objects that comprise both the tab panel and its button.
I recommend writing a separate function for irresolute tabs. You can either shop the previously selected tab and change only the styles needed to hide that and testify the new 1, or you can just update the fashion of all tabs every fourth dimension a new tab is selected.
Yous might want to telephone call this function immediately to make the interface start with the first tab visible.
What Is The Event Handler? How Do I Register/remove The Event Handler For The Node?,
Source: https://eloquentjavascript.net/15_event.html
Posted by: kelseyupolkinsuct.blogspot.com
0 Response to "What Is The Event Handler? How Do I Register/remove The Event Handler For The Node?"
Post a Comment